Documentation
Getting started with JxBrowser
This guide shows how to start working with JxBrowser in your Gradle or Maven project as quickly as possible.
Prerequisites
Please make sure your system meets the software and hardware requirements.
Choose your project build tool
Please add the JxBrowser Gradle plugin to your build script file build.gradle(.kts)
and configure the JxBrowser dependency as shown below:
plugins {
id 'com.teamdev.jxbrowser' version '1.2.1'
}
jxbrowser {
version '7.41.3'
}
dependencies {
implementation jxbrowser.crossPlatform
}
plugins {
id("com.teamdev.jxbrowser") version "1.2.1"
}
jxbrowser {
version = "7.41.3"
}
dependencies {
implementation(jxbrowser.crossPlatform)
}
Please add JxBrowser to your pom.xml
as shown below:
<repositories> <repository> <id>com.teamdev</id> <url>https://europe-maven.pkg.dev/jxbrowser/releases</url> </repository> </repositories> <dependencies> <dependency> <groupId>com.teamdev.jxbrowser</groupId> <artifactId>jxbrowser-cross-platform</artifactId> <version>7.41.3</version> <type>pom</type> </dependency> </dependencies>
Choose the software you are/will be working on
The following simple example demonstrates how to load a web page, wait until it's loaded completely, and print its HTML:
// Initialize Chromium.
EngineOptions options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build();
Engine engine = Engine.newInstance(options);
// Create a Browser instance.
Browser browser = engine.newBrowser();
// Load a web page and wait until it is loaded completely.
browser.navigation().loadUrlAndWait("https://html5test.teamdev.com/");
// Print HTML of the loaded web page.
browser.mainFrame().ifPresent(frame -> System.out.println(frame.html()));
// Shutdown Chromium and release allocated resources.
engine.close();
// Initialize Chromium.
val options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build()
val engine = Engine.newInstance(options)
// Create a Browser instance.
val browser = engine.newBrowser()
// Load a web page and wait until it is loaded completely.
browser.navigation().loadUrlAndWait("https://html5test.teamdev.com/")
// Print HTML of the loaded web page.
browser.mainFrame().ifPresent { frame -> println(frame.html()) }
// Shutdown Chromium and release allocated resources.
engine.close()
See full example on GitHub.
Choose your Java UI toolkit
Please add the JxBrowser Swing library to your build.gradle
:
dependencies {
implementation jxbrowser.swing
}
dependencies {
implementation(jxbrowser.swing)
}
Please add the JxBrowser Swing library to your pom.xml
:
<dependency> <groupId>com.teamdev.jxbrowser</groupId> <artifactId>jxbrowser-swing</artifactId> <version>7.41.3</version> </dependency>
Now you can embed JxBrowser Swing component into your Java Swing application as shown below:
// Initialize Chromium.
EngineOptions options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build();
Engine engine = Engine.newInstance(options);
// Create a Browser instance.
Browser browser = engine.newBrowser();
SwingUtilities.invokeLater(() -> {
JFrame frame = new JFrame("JxBrowser AWT/Swing");
frame.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
// Shutdown Chromium and release allocated resources.
engine.close();
}
});
// Create and embed Swing BrowserView component to display web content.
frame.add(BrowserView.newInstance(browser));
frame.setSize(1280, 800);
frame.setLocationRelativeTo(null);
frame.setVisible(true);
// Load the required web page.
browser.navigation().loadUrl("https://html5test.teamdev.com/");
});
// Initialize Chromium.
val options = EngineOptions.newBuilder(RenderingMode.HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build()
val engine = Engine.newInstance(options)
// Create a Browser instance.
val browser = engine.newBrowser()
SwingUtilities.invokeLater {
val frame = JFrame("JxBrowser AWT/Swing")
frame.addWindowListener(object: WindowAdapter() {
override fun windowClosing(e: WindowEvent) {
// Shutdown Chromium and release allocated resources.
engine.close()
}
})
// Create and embed Swing BrowserView component to display web content.
frame.add(BrowserView.newInstance(browser))
frame.setSize(1280, 800)
frame.setLocationRelativeTo(null)
frame.isVisible = true
// Load the required web page.
browser.navigation().loadUrl("https://html5test.teamdev.com/")
}
You should see the following output:
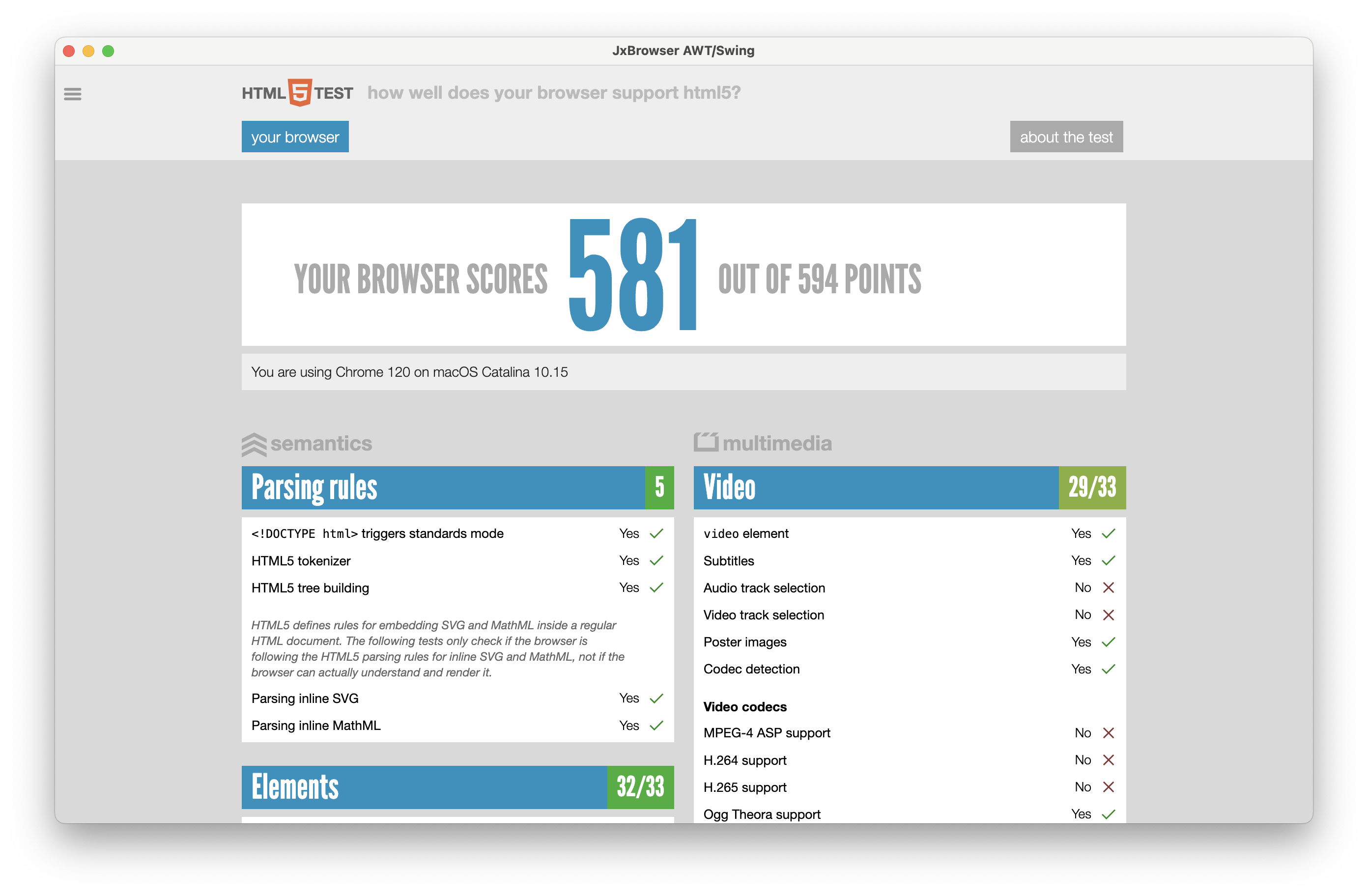
Please feel free to download and use an already configured project with Swing GUI from GitHub.
Please add the JxBrowser JavaFX library to your build.gradle
:
dependencies {
implementation jxbrowser.javafx
}
dependencies {
implementation(jxbrowser.javafx)
}
Please add the JxBrowser JavaFX library to your pom.xml
:
<dependency> <groupId>com.teamdev.jxbrowser</groupId> <artifactId>jxbrowser-javafx</artifactId> <version>7.41.3</version> </dependency>
Now you can embed JxBrowser JavaFX control into your JavaFX application as shown below:
// Initialize Chromium.
EngineOptions options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build();
Engine engine = Engine.newInstance(options);
// Create a Browser instance.
Browser browser = engine.newBrowser();
// Load the required web page.
browser.navigation().loadUrl("https://html5test.teamdev.com");
// Create and embed JavaFX BrowserView component to display web content.
BrowserView view = BrowserView.newInstance(browser);
Scene scene = new Scene(new BorderPane(view), 1280, 800);
primaryStage.setTitle("JxBrowser JavaFX");
primaryStage.setScene(scene);
primaryStage.show();
// Shutdown Chromium and release allocated resources.
primaryStage.setOnCloseRequest(event -> engine.close());
// Initialize Chromium.
val options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build()
val engine = Engine.newInstance(options)
// Create a Browser instance.
val browser = engine.newBrowser()
// Load the required web page.
browser.navigation().loadUrl("https://html5test.teamdev.com")
// Create and embed JavaFX BrowserView component to display web content.
val view = BrowserView.newInstance(browser)
val scene = Scene(BorderPane(view), 1280.0, 800.0)
primaryStage.setTitle("JxBrowser JavaFX")
primaryStage.setScene(scene)
primaryStage.show()
// Shutdown Chromium and release allocated resources.
primaryStage.setOnCloseRequest { engine.close() }
You should see the following output:
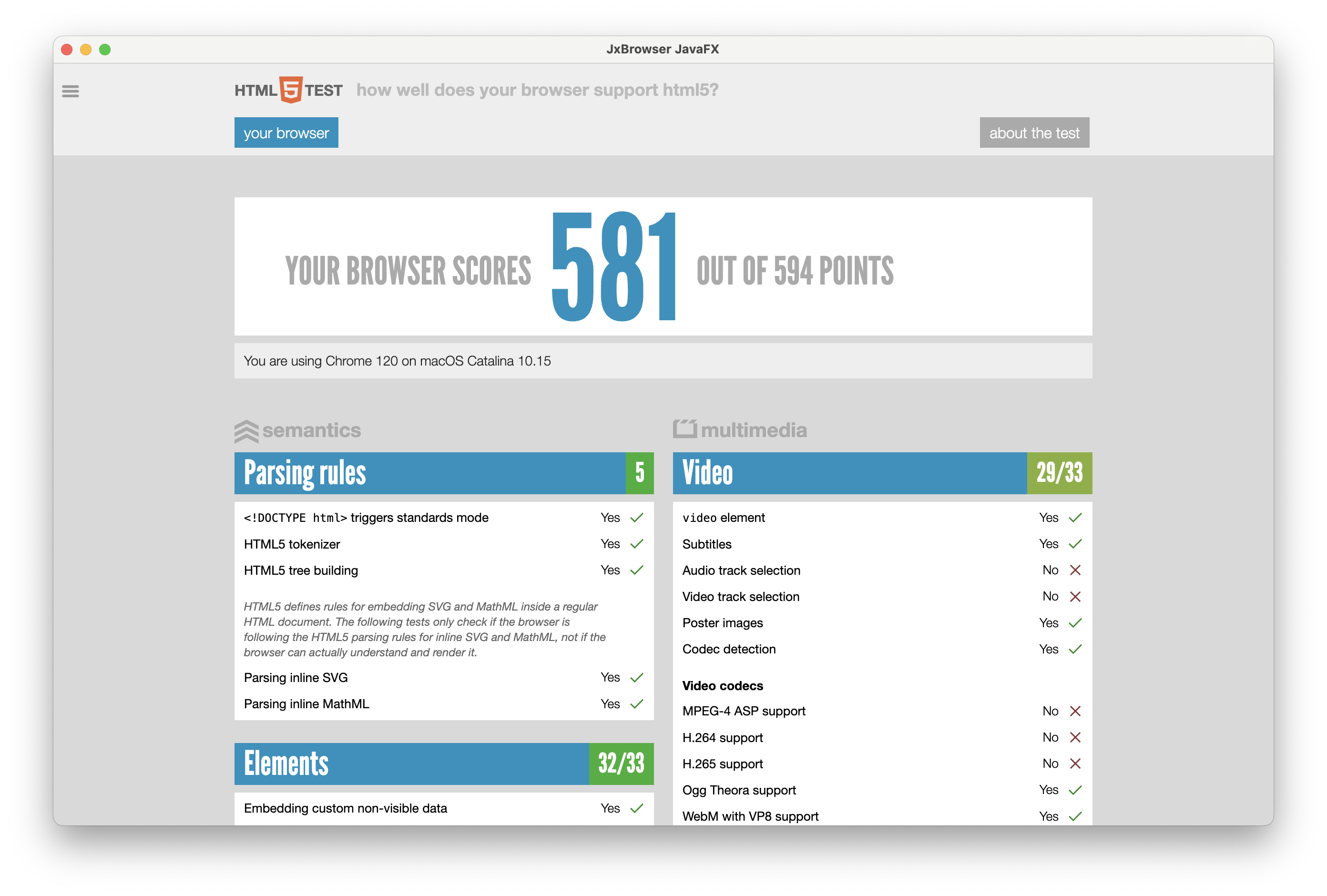
Please feel free to download and use an already configured project with JavaFX GUI from GitHub.
Please add the JxBrowser SWT library to your build.gradle
:
dependencies {
implementation jxbrowser.swt
}
dependencies {
implementation(jxbrowser.swt)
}
Please add the JxBrowser SWT library to your pom.xml
:
<dependency> <groupId>com.teamdev.jxbrowser</groupId> <artifactId>jxbrowser-swt</artifactId> <version>7.41.3</version> </dependency>
Now you can embed JxBrowser SWT widget into your Java SWT application as shown below:
// Initialize Chromium.
EngineOptions options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build();
Engine engine = Engine.newInstance(options);
// Create a Browser instance.
Browser browser = engine.newBrowser();
// Load the required web page.
browser.navigation().loadUrl("https://html5test.teamdev.com");
Display display = new Display();
Shell shell = new Shell(display);
shell.setText("JxBrowser SWT");
shell.setLayout(new FillLayout());
// Create and embed SWT BrowserView widget to display web content.
BrowserView view = BrowserView.newInstance(shell, browser);
view.setSize(1280, 800);
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
// Shutdown Chromium and release allocated resources.
engine.close();
display.dispose();
// Initialize Chromium.
val options = EngineOptions.newBuilder(HARDWARE_ACCELERATED)
.licenseKey("your license key")
.build()
val engine = Engine.newInstance(options)
// Create a Browser instance.
val browser = engine.newBrowser()
// Load the required web page.
browser.navigation().loadUrl("https://html5test.teamdev.com")
val display = Display()
val shell = Shell(display)
shell.setText("JxBrowser SWT")
shell.setLayout(FillLayout())
// Create and embed SWT BrowserView widget to display web content.
val view = BrowserView.newInstance(shell, browser)
view.setSize(1280, 800)
shell.pack()
shell.open()
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep()
}
}
// Shutdown Chromium and release allocated resources.
engine.close()
display.dispose()
You should see the following output:
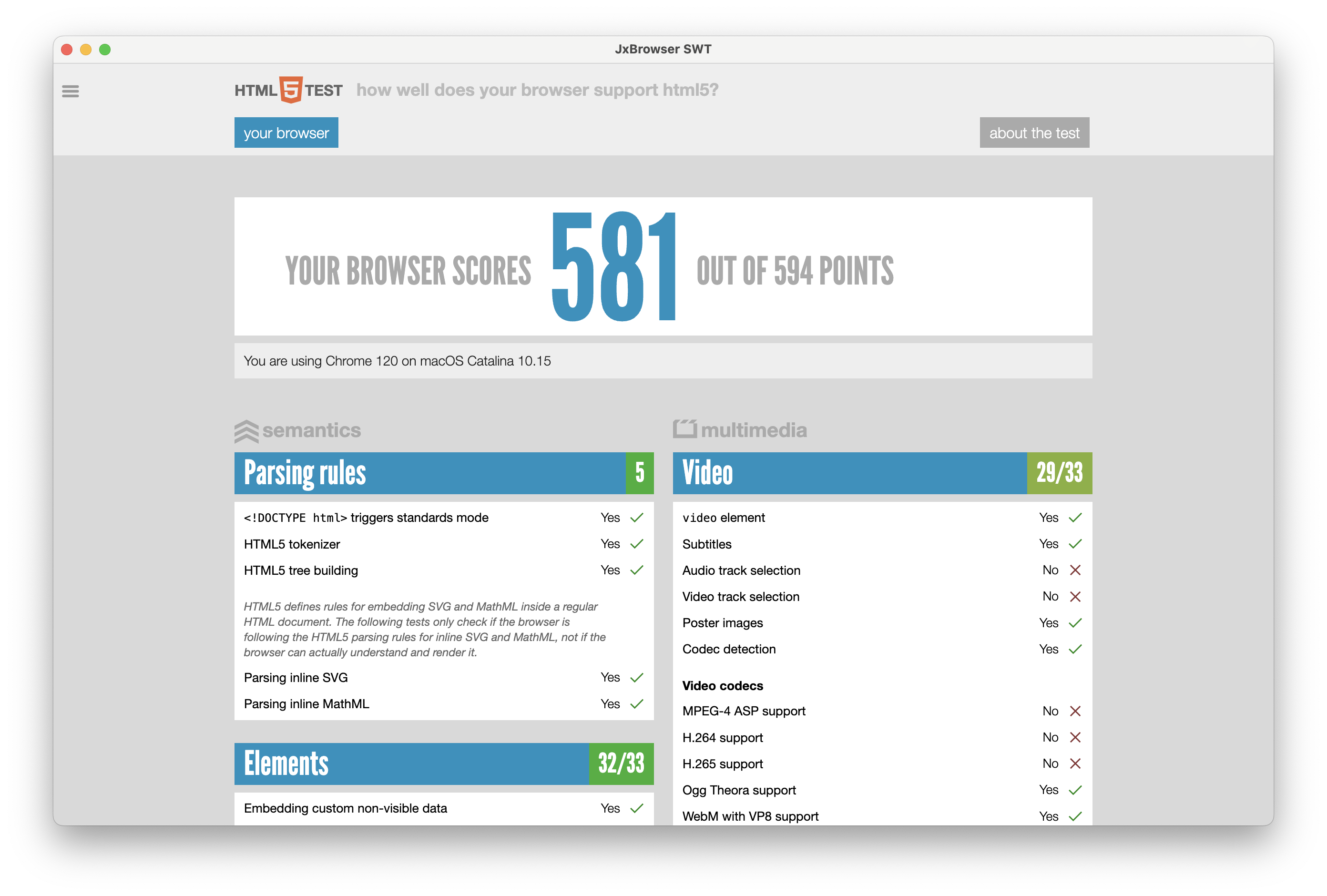
Please feel free to download and use an already configured project with SWT GUI from GitHub.
What's next
- Read how JxBrowser works in the architecture and design guides.
- Check out our guides to learn more about all JxBrowser features.
- Explore the JxBrowser API in reference documentation.